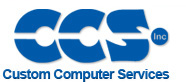 |
 |
View previous topic :: View next topic |
Author |
Message |
mictel
Joined: 24 May 2014 Posts: 17
|
Manual PWM set up works but #use pwm() does not; Why? |
Posted: Fri Mar 07, 2025 10:59 pm |
|
|
Working with PIC12LF1822 with PWM application and have a couple questions. First, Manual CCP1 set up generates PWM output on re-mapped pin (A5) but duty cycle is not as expected, not sure why. Duty cycle value 0x200 should be 50% but results in 64%. Have to use value 0x190 for 50% duty cycle. Here is the code. PCW latest version: 5.118
Code: | #include <12LF1822.h>
#fuses NOMCLR INTRC_IO NOWDT, BROWNOUT, PUT
#use delay(clock=2000000)
void main() {
setup_oscillator(OSC_2MHZ); // Set internal oscillator to 2MHz
setup_ccp1(CCP_PWM | CCP_P1A_A5); // Output on pin A5 (default is A2)
setup_timer_2(T2_DIV_BY_1, 199, 1); // Set PWM frequency to 2.5KHz with 10-bit resolution
set_pwm1_duty(0x190); // Set pwm1 duty cycle; duty cycle = value / [4* (Timer Period + 1)]
// value = (duty cycle)*4*(Timer Period +1)
// value = (50)*4*(2000000 +1) = 0x200 10 bit value
// BUT value=0x200 results in duty cycle of 64% NOT 50%
// value = 0x190 results in duty cycle 50%, WHY????
// Main Loop
while(TRUE){
delay_ms(10); // Wait 10 ms
}
} |
Second, I would much rather use the more compact and easier compiler directive #use pwm() set up but cannot get it to work. It compiles fine and lists the pwm bit resolution (9.64 bits) 0 Errors and 0 Warnings BUT no output on pin A5. Why? I tried several options without success I found a similar thread discussing this issue that suggested it might be a bug in earlier compiler version so I updated to the latest PCW version and still NG. Thank You for reviewing.
Code: | #include <12LF1822.h>
#fuses NOMCLR INTRC_IO NOWDT, BROWNOUT, PUT
#use delay(clock=2000000)
#use PWM(PWM1, OUTPUT=PIN_A5, TIMER=2, FREQUENCY=2500, DUTY=50)
void main() {
pwm_on();
// Main Loop
while(TRUE){
pwm_on();
delay_ms(10); // Wait 10 ms
}
}
|
Code: | Compiling C:\Users\Documents\pwm_not_working_main on 07-Mar-25 at 23:47
--- Info 300 "C:\Users\Documents\HAM\pwm_not_working_main.c" Line 4(1,1): More info: PWM Resolution: 9.64 bits
Memory usage: ROM=3% RAM=4% - 13%
0 Errors, 0 Warnings.
Build Successful.
CCSLOAD: Connecting
CCSLOAD: Programming PIC12LF1822
CCSLOAD: Programming Complete
CCSLOAD: Target Running at 23:47
|
|
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19730
|
|
Posted: Sat Mar 08, 2025 5:04 am |
|
|
On the duty cycle, your calculations are wrong. 199 for the PR2, means the
PWM counts 0 to 799. So 50% would be 399/400 0x18F/0x190. Exactly what
you are seeing......
Remember the PWM counts to ((PR2+1)*4)-1, and you are specifying the
PR2 value in decimal not hex.
On the A5, the problem here is that this requires setting the internal control
bit to select the alternate pin. The setup_ccp1 function 'knows' about this,
but the #use PWM function only knows about the primary pin.
Code: |
#include <12LF1822.h>
#fuses NOMCLR INTRC_IO NOWDT, BROWNOUT, PUT
#use delay(INTERNAL=2000000)
#use PWM(PWM1, OUTPUT=PIN_A2, TIMER=2, FREQUENCY=2500, DUTY=50)
#bit CCPISEL=getenv("BIT:CCP1SEL")
void main() {
CCPISEL=1; //Now the CCP goes to pin A5
|
Note also how to setup the clock. |
|
 |
mictel
Joined: 24 May 2014 Posts: 17
|
|
Posted: Sat Mar 08, 2025 12:18 pm |
|
|
Ttelmah, a sincere thank you for your prompt review and for answering my questions. Rookie misunderstanding the PWM duty cycle calculation.
Regarding the #use pwm directive; it seems the directive is not fully implemented OR the help file is incorrect.
From the Help File:
#use pwm()
Syntax:
#use pwm (options)
Elements:
option - may be any of the following separated by a comma:
PWMx or CCPx - Selects the CCP to use, x being the module to use.
OUTPUT=PIN_xx - Selects the PWM pin to use, pin must be one of the CCP pins. If device has remappable pins compiler will assign specified pin to specified CCP module. If CCP module not specified it will assign remappable pin to first available module.
Which it does not. Much appreciated and very thankful for you and the other subject matter experts here who share their knowledge and experience here for the benefit of others. |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19730
|
|
Posted: Sat Mar 08, 2025 12:27 pm |
|
|
It is a question of detail in the wording.
This chip has single alternate pins, not 'remappable' pins.
The manual is talking about chips that support PPS, and can map the
pins to a lot of locations. These use #pin select.
The pity is that the manual does not make this distinction.
The sticky at the top of the forum explains the three different ways
pins are moved, but the manual does not have any syntax to distinguish
them.  |
|
 |
mictel
Joined: 24 May 2014 Posts: 17
|
|
Posted: Sat Mar 08, 2025 12:53 pm |
|
|
Revised code but still no pwm output on alternate pin A5.
Had to add one more control statement to get it to work: output_drive(PIN_A5); Learned this from previous post by PCM Programmer, thank you.
Sure would be nice if the the #use pwm directive worked as documented to take care of all the background set up. Will alert CCS
Code: | #include <12LF1822.h>
#fuses NOMCLR INTRC_IO NOWDT, BROWNOUT, PUT
#use delay(clock=2000000)
#use PWM(PWM1, OUTPUT=PIN_A2, TIMER=2, FREQUENCY=2500, DUTY=50)
#bit CCPISEL=getenv("BIT:CCP1SEL") //Alternate Pin Function Control (AFPCON) register for CCP1 output mapping
// CCPISEL=0; pwm output on Pin A2 (default)
// CCPISEL=1; pwm output on alternate Pin A5
void main() {
CCPISEL=1; // remap pwm output to alternate pin A5
output_drive(PIN_A5); // also required to re-map pwm from A2 to A5
// Main Loop
while(TRUE){
pwm_on(); // enable pwm output
delay_ms(100); // Wait 100 ms
pwm_off(); // disable pwm output
delay_ms(100); // Wait 100 ms
}
}
|
|
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19730
|
|
Posted: Sat Mar 08, 2025 1:25 pm |
|
|
Yes. This sets the TRIS. By default the pin is an input. Forgot to say you
needed to set the tris.
I must admit I think it might be worth asking CCS if they could add support
for APFCON to the #USE PWM setup. Would then get rid of this fiddle.... |
|
 |
Ttelmah
Joined: 11 Mar 2010 Posts: 19730
|
|
Posted: Sun Mar 09, 2025 3:03 am |
|
|
I have emailed support pointing this out, and suggesting that they should
either include support for APFCON in the #USE operation, or amend the
manual to say that the relocatable pin support is only for PPS chips.
Best Wishes |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB © 2001, 2005 phpBB Group
|